Builder > Custom code
You can add javascript code in your dialog flows using the Bot does block.
Drag and drop the block and connect it to the state where you want to see executed.
You can then add your own code, it will be hosted and executed in the secure and scalable servers of the bot platform.
Warning : If you use a "bot does" after a "bot shows", the bot does will be prioritarty
Embedded Code Editor
You can then double click on the block to open the integrated code editor.
You can see some commented code snippets that may be helpful.
We currently offer support for Node.js, additional languages may be available in the future.
The code editor comes with a dark mode.
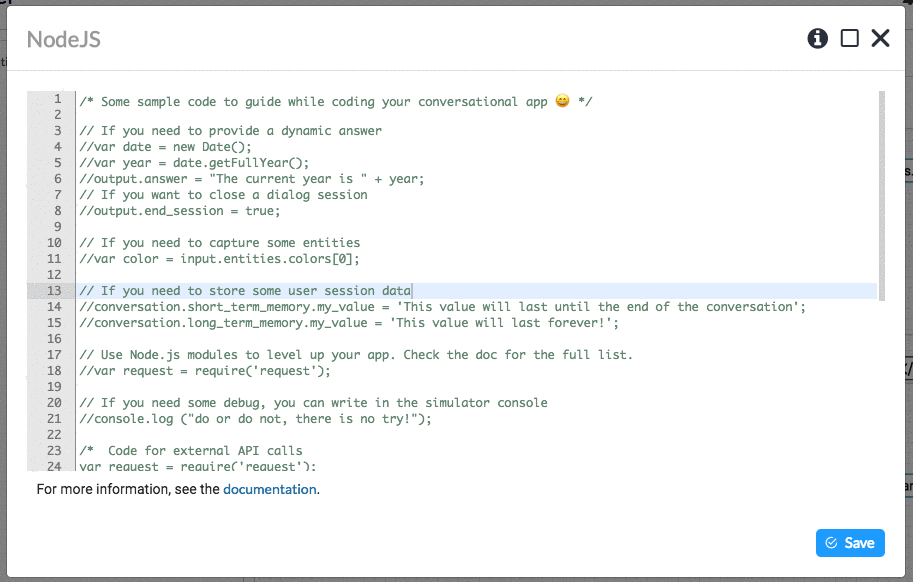
The code editor has a dark mode 🌓
What can I do with the Node.js?
- Create a dynamic answer
- Access entities and contextual values
- Send requests to your preferred APIs and databases
- Control connected devices
- Implement business logic or complex algorithms
- ...and much much more!
Is there any limitation to what I can put in my code?
While coding your business logic, please keep two things in mind:
- In the case your code takes time to be fully executed, please keep in mind that you have a 90 seconds limit, after that, beyond that limit, the code execution will be stopped and your bot will return a timeout.
- Always have the `callback(output)``in your code, wich indicates to the platform that your custom code has been fully executed.
callback(output); // 👈 Never Forget this callback
Can I keep some contextual data?
Yes you can.
Here is how you can store data as conversation context
conversation.shared_memory.my_new_field = 'my_value';
Shared memory
If you are using the main bots / micro bots architecture, the data stored in the
conversation.shared_memory
will be available across all the microbots.
How can I leverage user data to create more personal experiences ?
If some user data has been sent to the bot platform, you may access it by code using the following syntax:
var first_name = user_data.first_name;
Can I get the user input?
Sure! Here is the code to so:
var user_message = input.text;
Can I get interesting entities from the user input?
Sure, that's the purpose of custom and system entities.
Let's see how you can do that.
- Custom entities
Assuming, you have created an entity calledcolors
, and added it to an intent that has been detected,. You can get your custom entity using the following syntax:
var color = input.custom_entities.colors[0].value;
- System entities
The platform offers pre-trained entities that you can capture anytime using the appropriate syntax.
Also, please check the system entity availability in the Language Support page.
// time: today, Monday, Feb 18, the 1st of march
var time = input.system_entities.time[0].value;
// temperature: 70°, 72° Fahrenheit, thirty two celsius
var duration = input.system_entities.duration[0].value;
// number: eighteen, 0.77, 100K
var number = input.system_entities.number[0].value;
// amount of money: ten dollars, 4 bucks, $20
var amount_of_money = input.system_entities.amount_of_money[0].value;
// duration: 2 hours, 4 days, 3 minutes
var duration = input.system_entities.duration[0].value;
// email: [email protected]
var email = input.system_entities.number[0].value;
// url: https://smartly.ai, www.google.com
var url = input.system_entities.url[0].value;
// phone number: 012345678
var phone_number = input.system_entities["phone-number"][0].value;
You can change the editor’s theme to dark and also make the editor full screen.
How can I make API calls from the bot to my information system safer?
Leveraging your webservices can unleash valuable use cases to your users.
Here is a list of things you can do to keep a good level of security while connecting your bot to your IS via public APIs:
- Use a public key in your API calls
- Use a private key for the bot and change it at least once a month
- Use a dedicated and expirable access token for each user
- Use IP filtering to restrict the access to your API to the following adresses: Bot Platform IP adresses
What version of Node.js do I get?
Current version of Node.js you can use in your bots is 18.15.0
Hydrogen.
Is there any pre-installed packages that I can use?
The following packages have been pre-installed.
You can use them directly in your bot projects.
{
"algoliasearch": "5.13.0",
"async": "2.1.5",
"axios": "1.7.7",
"cheerio": "1.0.0",
"crypto-js": "3.1.9-1",
"debug": "2.6.1",
"docdash": "0.4.0",
"fs": "0.0.1-security",
"github": "9.0.0",
"google-auth-library": "8.6.0",
"googleapis": "16.1.0",
"htmlparser2": "3.9.2",
"http": "0.0.0",
"https": "1.0.0",
"jsforce": "1.10.1",
"jsonschema": "1.4.1",
"jsrender": "1.0.5",
"lodash": "4.17.4",
"mathjs": "3.9.2",
"md5": "2.2.1",
"moment": "2.19.1",
"moment-timezone": "0.5.14",
"mqtt": "2.18.3",
"mysql": "2.17.1",
"node-salesforce": "0.8.0",
"nodemailer": "3.1.3",
"nodemailer-smtp-transport": "2.7.2",
"oauth": "0.9.15",
"puppeteer": "23.7.1",
"purecloud_api_sdk_javascript": "0.84.8",
"purecloud-platform-client-v2": "69.2.1",
"querystring": "0.2.0",
"request": "2.87.0",
"restify": "4.3.0",
"run-parallel": "1.1.6",
"serpapi": "2.1.0",
"sequence": "3.0.0",
"soap": "0.29.0",
"sync": "0.2.5",
"underscore": "1.8.3",
"url": "0.11.0",
"util": "0.10.3",
"uuid": "3.0.1",
"webcom": "2.8.0",
"winston": "3.3.3",
"xml": "1.0.1",
"xml2js": "0.4.17",
"yahoo-weather": "2.2.2"
}
As usual in Node, simply add a require('package name')
at the beginning of your code before using the package.
//if you want to use the mysql package
var mysql = require ('mysql');
Need a specific package?
If you need a specific package for your app, send us a request at [email protected] and our team will review it.
Send data from the bot to the client via the API
You can send some data using our API using the payload
field
output.payload.my_value = "my value";
You can send any data using the payload
field 🙂
Jump to bot
In some cases you may want to jump from a bot to another, here is more details on how to implement this behavior.
Jump to bot documentation
Updated 7 months ago